Introduction
Loops are fundamental building blocks in JavaScript, allowing you to execute code repeatedly. Choosing the right loop type for the task at hand is crucial for writing efficient, clean, and maintainable code.
This guide explores various loop types in JavaScript, their ideal use cases, and practical optimization tips to elevate your coding skills.
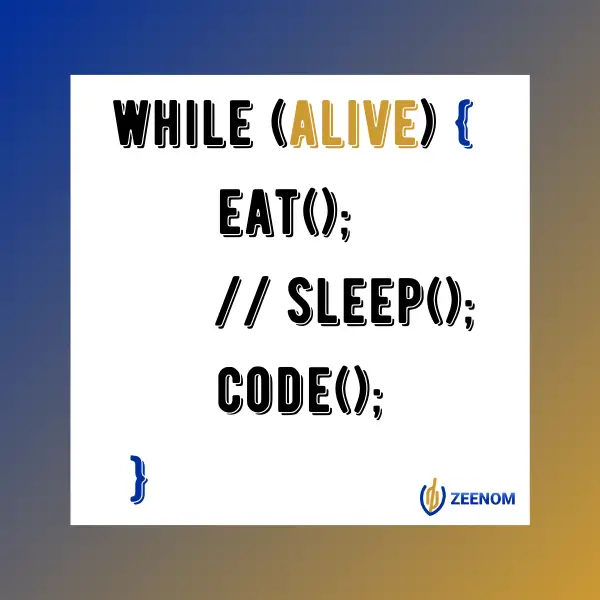
Exploring Loop Types
JavaScript offers a versatile toolbox of loop constructs, each with its strengths and applications:
π for…of Loop
Ideal for iterating over iterable objects (like arrays) when you only need the values. It’s concise and easy to read, making it perfect for simple iterations.
const products= ["iphone", "Samsumg", "Redmi"]
for (const item of products){
console.log(item)
}
// Output: iphone, Samsumg, Redmi
π for Loop
Grants full control over loop conditions, making it ideal for working with arrays where you need both the item value and its position (index).
const products= ["Mouse", "Keyboard", "Laptop", "iPhone"]
for (let i = 0; i < products.length; i++){
console.log(products[i])
}
// Output: Mouse, Keyboard, Laptop, iPhone
π for…in Loop
Designed for looping through object properties. Use this loop when you need to display key-value pairs or modify properties dynamically.
const user = {}; // Initially empty object
// Add properties dynamically
user.name = "Roland";
user.age = 29;
user["is_admin"] = true;
for (const key in user) {
console.log(key, user[key]); // Access value using key
}
// Output: name Roland, age 29, is_admin true
π forEach Method
A functional approach to iterating over arrays. It provides a cleaner syntax for applying a function to each item. Limited control, cannot use break or continue statements.
products.forEach(item => console.log(item));
// Output: iphone, Samsumg, Redmi
π map Method
For transforming each item in an array and creating a new array with the results. It’s concise and functional, perfect for data manipulation.
const numbers = [1, 2, 3];
const doubledNumbers = numbers.map(number => number * 2);
// Output: doubledNumbers: [2, 4, 6]
π filter Method
Creates a new array with elements that pass a specific condition. It’s only useful for filtering, not for general iterations.
const users = [
{ active: true },
{ active: false },
{ active: true },
];
const activeUsers = users.filter(user => user.active);
// Output:
activeUsers: [{ active: true }, { active: true }]
π reduce Method
A powerful tool for aggregating values in an array into a single result. It’s particularly useful for calculations or combining elements.
const numbers = [1, 2, 3];
const sum = numbers.reduce((accumulator, number) => accumulator + number, 0);
// Output:
sum: 6
Choosing the Right Loop for the Job
Now that you’re familiar with the core loop types, let’s explore when to use each one effectively:
- Simple Iteration: Utilize the clean and efficient for…of loop for basic array or string iterations.
- Need Item Positions? Employ the traditional for loop or the functional forEach method when you require access to both item values and their positions within the collection.
- Object Property Loops: Rely on the for…in loop to traverse object properties. Remember to be cautious of inherited properties from the prototype chain.
- Functional Transformations: Leverage the power of map and filter methods for transforming or filtering array elements. These methods create new arrays based on your operations.
- Aggregation Tasks: The reduce method is your champion for accumulating values into a single result. It’s particularly useful for calculations or combining elements.
Optimizing Your Loops for Performance
While loops are powerful, understanding their performance implications is essential. Here are some optimization tips to keep in mind:
- Performance Considerations: For large datasets, methods like map and filter can outperform traditional for loops due to their internal optimizations.
- Error Handling: Be mindful of potential errors. Watch out for off-by-one mistakes in for loops and unintended modifications to prototype properties with for…in loops.
Loop Summary Table
Loop Type | Best For | Pros | Cons |
---|---|---|---|
for…of | Iterating over iterable objects (arrays, strings) for values | Simple, concise, good for basic iterations | No access to item positions (index) |
for | Full control over loop conditions, especially for arrays | Access to both item positions (index) and values | More setup required, potential for off-by-one errors |
for…in | Looping over object properties | Easy access to object properties | Iterates over inherited properties as well, use with caution |
forEach | Neatly iterating over arrays, applying a function to each item | Clean and functional syntax | Limited control, cannot use break or continue statements |
map | Transforming each item in an array and creating a new array with the results | Concise and functional for data manipulation | Not suitable for simple iterations without value transformation |
filter | Creating a new array with items that meet a specific condition | Functional for filtering unwanted items | Only useful for filtering, not for general iterations |
reduce | Aggregating values in an array into a single result | Powerful for calculations or combining elements | Complex for simple iterations, requires careful handling |
By mastering these looping techniques and understanding their strengths and weaknesses, you’ll be well-equipped to tackle various coding challenges efficiently.
For a deeper understanding of JavaScript loops, check out this comprehensive guide on MDN Web Docs
Share Your Thoughts!
Which JavaScript looping method do you find most useful in your projects? Share your experiences, challenges, and insights in the comments below! Letβs learn and grow together.
Stay tuned for more coding insights and practical tips!